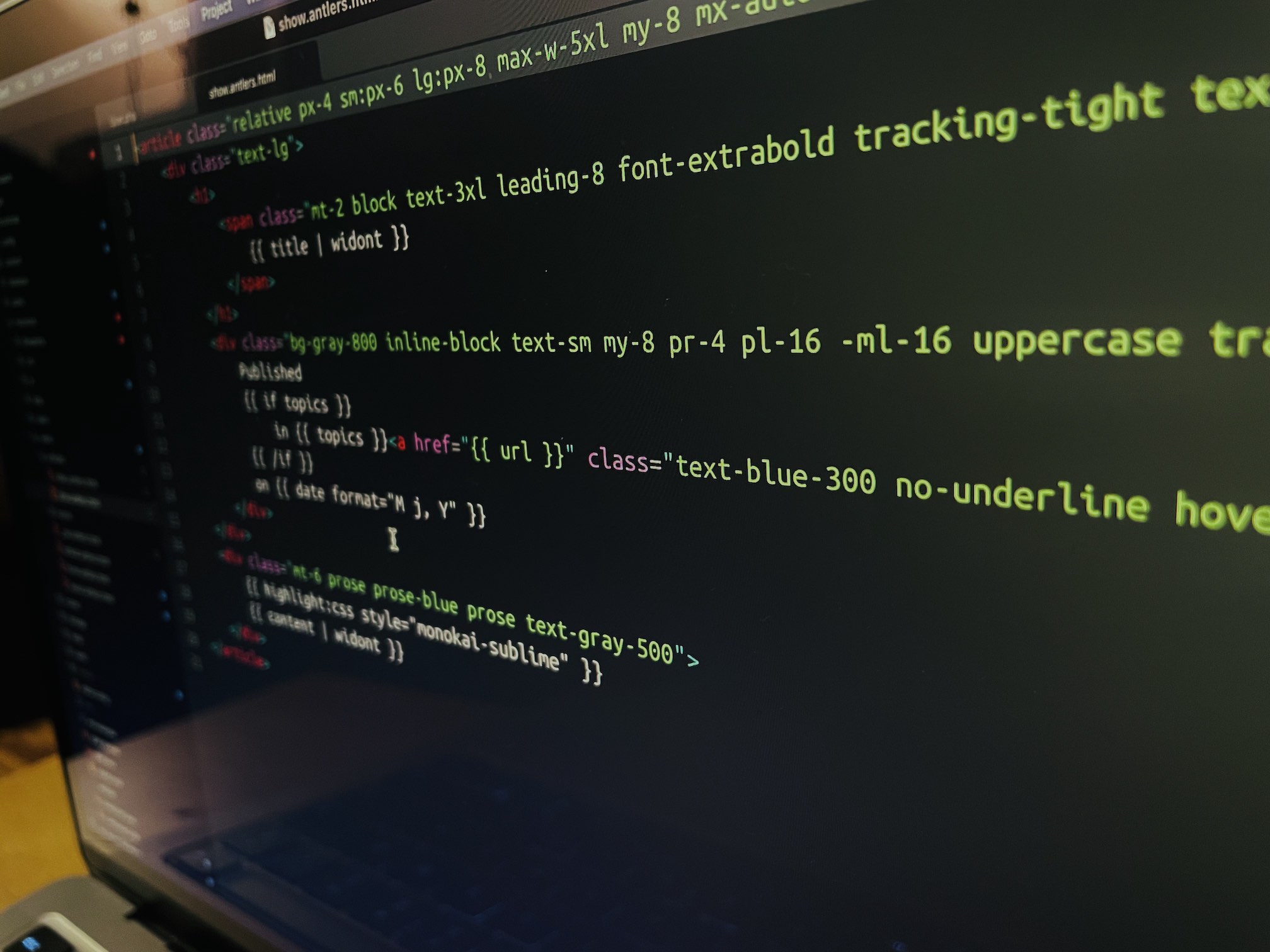
Sublime Text 4 is finally out and it's time to go back to your favorite text editor. I will give you a short overview of what's new with Sublime Text and go over the differences with PHPStorm and VSCode (VIM users are not the target audience, sorry Michael Dyrynda 🤷♂️).
What's new?
- It's fast. It's even faster than before with hardware acceleration and support for M1.
- It finally has code intelligence (called context-aware auto-complete) and with that, first class support for language servers (LSP).
- It has a new license model: Buy a license, get 3 years of updates.
- It as a new name: Sublime Text, no version number. With the new license model, Sublime Text will now continuously receive updates, so no more need for major releases.
- It has an amazing new default theme, light and dark, so no need to fight! Dark is better, though.
- It has full TypeScript support!
- It has an easy way to display files in split panes called Tab Multi Select that is integrated in a dozen places.
To see the features illustrated, check out this overview video.
Why try it (or not)?
If you're a full-blown IDE person and cannot live without all those nice refactoring features in your day to day work, you're probably going to stay with PHPStorm. If you're good with what Intelliphense has to offer plus niceties like automatically adding use statements or expanding FQCNs, Sublime Text is for you.
For VSCode users the only downside seems to be that ST4 is not free. Other than that you will see the same level of code completion capabilities and a way snappier editor.
How I use Sublime Text for Laravel Development
Before I go into the details of how I set ST up exactly, I want to write a little about how I use it and its features on a daily basis.
Testing
I practice TDD whenever it makes sense, so the first thing is usually do is generate a functional test. In that test-file I just type verbatim text of what I want to test (i.e. "it displays a list of blog posts"). Pressing ctrl+e converts this text to a proper test method that I just need to fill with life.
To run tests, I just press ctrl+shift+n
(nearest) while the cursor is inside the method I want to test. ctrl+shift+l
(last) will repeat the last test run.
Creating things
For files that are not generated by artisan, I press Cmd+Shift+n
to create a new file. I can use tab-autocompletion to navigate folders from the project root or start the filename with a colon (:) to create the file in the directory of the currently open file.
As soon as I have an existing file, I usually use snippets (linked below) to create code structures. _c
will create a class (getting the classname from the filename). The PHP Companion plugin helps adding the correct namespace (I use F6
for this).
The snippets _f
and _pf
help creating public functions and protected functions respectively.
PHP Stuff
PHP Companion as some more nice features. It allows me to easily class/constructor properties by pressing F7
and just typing the name.
When using PHP classes, I press F10
to import the fully qualified classname as a use statement at the top of the file (automatically sorted). When implementing an interface or an abstract class, there's also the option to import all methods that need to be implemented. No extra shortcut, I just use the command palette (cmd+shift+p
).
Autocomplete
I'm not sure how autocomplete works without a language server, but the default in ST4 is already so good, that I haven't bothered with installing Intelliphense of Psalm, yet. I do use the Tailwind plugin to get all the Tailwind classes.
Linting
For linting I use phpcs and tlint by Tighten. Especially tlint has great linting for code quality (like unused imports). I've heard great things about Psalm, but haven't tried it with ST4, yet.
My setup for Laravel Development
Installation / Upgrade
ST4 will detect existing ST3 configuration files in ~/Library/Application Support/Sublime Text 3
(Mac) or ~/.config/sublime-text-3
(Linux). It will copy the existing config to the new location at ~/Library/Application Support/Sublime Text
or ~/.config/sublime-text
respectively. If you want to start fresh, just create an empty config directory.
If you haven't done so already, you should also install Sublime Merge, it works really nice in concert with Sublime Text (i.e. getting the history or blame for the current file).
You should also make sure the CLI is available for both tools, so that you can run subl
or smerge
in a terminal.
Plugins
Here's a list of all plugins I'm using.
- A File Icon, pretty icons for your files
- AceJump, quickly move the cursor to a specific position
- AdvancedNewFile, better creation of new files and renaming
- ChangeQuotes, change from double to single quotes or vice-versa
- EditorConfig, read and apply .editorconfig files
- Emmet, generate HTML plus tools to work with HTML (like wrap selection with tag)
- Laravel Blade Highlighter, add syntax for Laravel Blade templates
- Laravel Blade Spacer, automatically adds spaces to Laravel Blade syntax for readability
- PHP Companion, essential for PHP development
- PHP Completions Kit, provides PHP completions for Sublime Text
- PHP CS Fixer, code style fixer
- PHPUnitKit, run your PHPUnit tests in Sublime Texts console
- SublimeLinter, code linting
- SublimeLinter-contrib-tlint, support for tighten/tlint
- SublimeLimyer-php, lint PHP
- SublimeLinter-phpcs, lint code style problems
- Tailwind CSS Autocomplete
- Vue Syntax Highlight
This is what I used for ST3 and it works way better in ST4 because of the new context-aware code-completion. I haven't tried LSP plugins, yet, but have seen others using Intelliphense, Psalm, and the VSCode TailwindCSS Intellisense with great success.
Settings
Here's my commented settings file.
{
// prevent autocompletion on pressing ENTER
"auto_complete_commit_on_tab": true,
// don't be smart
"detect_indentation": false,
"folder_exclude_patterns":
[
".git",
".idea",
"node_modules",
"storage/framework"
],
"font_face": "Ubuntu Mono",
"font_size": 20,
"hide_tab_scrolling_buttons": true,
"hide_new_tab_button": true,
"highlight_line": true,
"highlight_modified_tabs": true,
// draw some lines to show me what indention level I'm in
"indent_guide_options": ["draw_normal", "draw_active"],
// this is important, ST4 indexer will ignore files in .gitignore by default
"index_exclude_gitignore": false,
"line_padding_bottom": 2,
"line_padding_top": 2,
"show_errors_inline": true,
"show_panel_on_build": true,
"tab_size": 4,
// use the new beautiful dark theme
"theme": "Default Dark.sublime-theme",
"ignored_packages":
[
"Vintage",
],
"show_tab_close_buttons": false,
"translate_tabs_to_spaces": true,
"trim_automatic_white_space": true,
"trim_trailing_white_space_on_save": true,
"word_wrap": false,
}
Package Settings
Some extra settings for the packages I use. These live in Packages/User
which you can find when you search the Command Palette for Browse Packages.
AdvancedNewFile.sublime-settings .
{
"rename_default": "<filepath>",
}
I add a file for the default theme to be able to quickly adjust the text-size of the interface (not the editor), to make it more legible when presenting. Just uncomment the two font lines, when you need to present to a remote audience.
Default Dark.sublime-theme .
{
"rules": [
{
"class": "sidebar_label",
// "font.face": "SF Pro Display",
// "font.size": 25,
}
],
}
Markdown.sublime-settings .
{
"trim_automatic_white_space": false,
"trim_trailing_white_space_on_save": false,
"word_wrap": true,
}
PHP Companion.sublime-settings .
{
// default visibility of class variables
"visibility": "public",
}
SublimeLinter.sublime-settings .
// SublimeLinter Settings - User
{
"linters":
{
"phpcs":
{
"@disable": false,
"args":
[
"--standard=PSR2",
"--exclude=Squiz.Scope.MethodScope,PSR1.Methods.CamelCapsMethodName"
],
"executable": "phpcs",
"excludes":
[
"*.html",
"*.blade.php"
]
},
"tlint":
{
"args": [],
"executable": "tlint"
}
},
"paths": {
"linux": ["~/.composer/vendor/bin"],
"osx": ["~/.composer/vendor/bin"],
"windows": []
},
}
Key Bindings
My non-default key bindings.
[
// add namespace
{ "keys": ["f6"], "command": "import_namespace" }
// add constructor property
{ "keys": ["f7"], "command": "insert_php_constructor_property" },
// add namespace to class (expand to fully qualified class name)
{ "keys": ["f9"], "command": "expand_fqcn" },
// add FQCN to use statements
{ "keys": ["f10"], "command": "find_use" },
// ace jump to a word
{ "keys": ["ctrl+,"], "command": "ace_jump_word" },
// test function closest to the cursor
{ "keys": ["ctrl+shift+n"], "command": "phpunit_test_nearest" },
// re-run last test
{ "keys": ["ctrl+shift+l"], "command": "phpunit_test_last" },
// convert sentence to test method
{ "keys": ["ctrl+e"], "command": "php_unit_test_method" },
// reveal current file in sidebar
{ "keys": ["super+shift+r"], "command": "reveal_in_side_bar"}
]
Snippets and Plugins
You can find my (very simple) snippets in this gist: Sublime Text Snippets.
The custom plugin that converts sentences to test methods is available in this gist: PHPUnitTestMethodPlugin.py. Some version of this plugin was written by Jeffrey Way but it has been modified since, not sure about the actual author.
Closing thoughts
In my opinion Sublime Text 4 is a joy to use as a daily driver. It probably won't replace your PHPStorm or VIM… but it might. If you have any comments, please reply to this tweet.